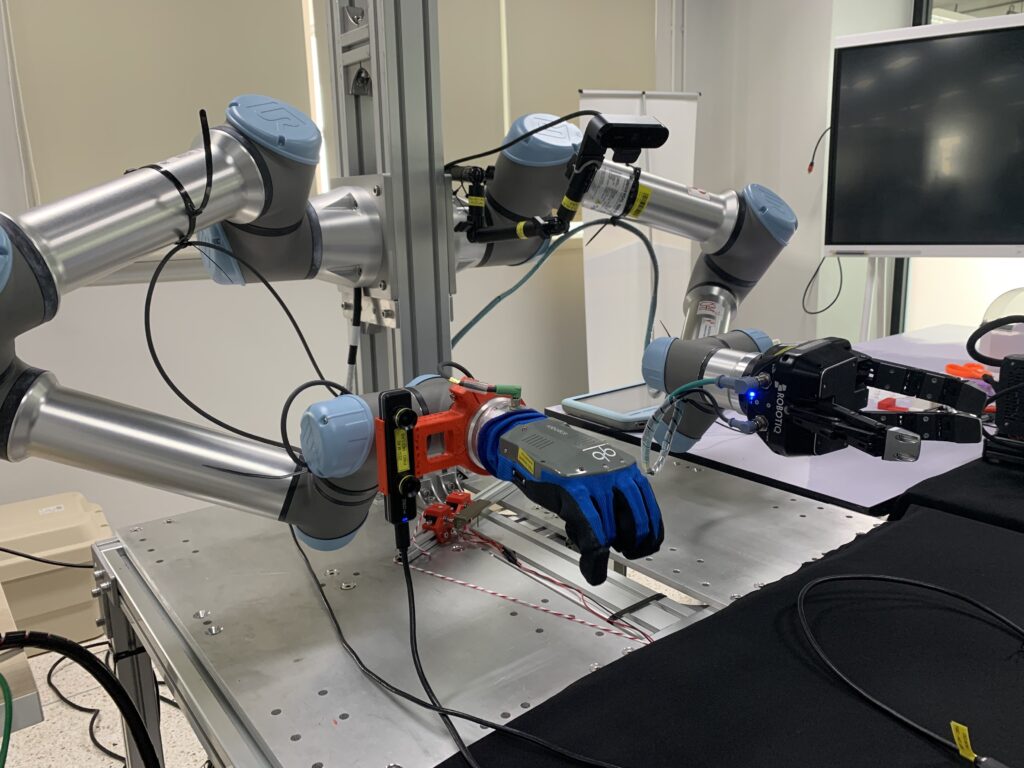
Communication between Python and UR5e
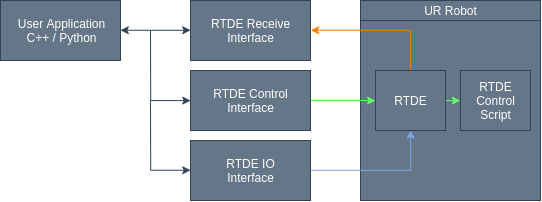
RTDE (Real-Time Data Exchange) เป็นโปรโตคอลที่ใช้สำหรับการสื่อสารแบบเรียลไทม์กับระบบหุ่นยนต์ของ Universal Robots (UR) อินเทอร์เฟซนี้ช่วยให้อุปกรณ์ภายนอกสามารถควบคุมและตรวจสอบหุ่นยนต์ UR ได้โดยการแลกเปลี่ยนข้อมูลด้วยความถี่สูง ซึ่งมักจะสูงถึง 500Hz
Key Components of UR RTDE
- RTDE Control Interface: Allows sending commands to the robot to control its movements and operations.
- RTDE Receive Interface: Used to receive data from the robot, such as sensor readings and status information.
ในส่วนของงานจะใช้ Python ในการสื่อสารหรือส่งคำสั่งไปยัง UR5e
ตัวอย่างของการเชื่อมต่อ Control_interface ด้วย TCP/IP (ต้องทำให้เครื่องคอมอยู่ในวงแลนเดียวกันกับ UR5e)
import rtde_control
rtde_c = rtde_control.RTDEControlInterface("127.0.0.1") #เปลี่ยน IP ให้อยู่ในวงเดียวกับ UR แต่ห้ามซ้ำกัน
rtde_c.moveJ_IK([-0.76585, -0.02775, 0.12318, 2.084, -0.096, -2.284], 0.5, 0.5) #HOME
rtde_c.moveL([-0.91579,0.19691,0.25434,2.341,-0.226,-3.536], 0.5, 0.5) #pick
ต่อมาเป็นตัวอย่างในการเชื่อมต่อ Receive_interface ด้วย TCP/IP เช่นกัน
import rtde_receive
rtde_r = rtde_receive.RTDEReceiveInterface("127.0.0.1") #เปลี่ยน IP ให้อยู่ในวงเดียวกับ UR แต่ห้ามซ้ำกัน
# Get current positions
actual_q = rtde_r.getActualQ()
actual_TCPpose = rtde_r.getActualTCPPose()
MQTT บน Python เพื่อใช้ในการส่งคำสั่ง
import paho.mqtt.client as mqtt
import time
# Define MQTT broker address and topics
broker_address = "10.7.147.120"
topic_command = "UR/control/robot"
# Create an MQTT client and connect to the broker
client = mqtt.Client()
client.connect(broker_address)
# Define a function to publish a robot command
def publish_command(robot_id, command, parameters):
command_str = ",".join([robot_id, command] + list(map(str, parameters)))
client.publish(topic_command, command_str)
#Publish command
publish_command("10", "movej", [0.73875, -0.04809, 0.12294, 2.431, -1.084, 2.588, 1.5, 1.5]) # HOME
client.loop_start()
try:
while True:
time.sleep(1)
except KeyboardInterrupt:
client.loop_stop()
client.disconnect()
STEAMING VIDEO
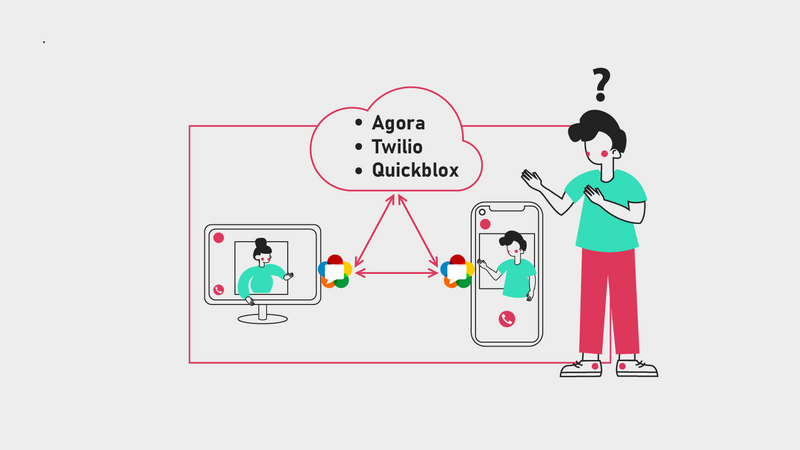
QuickBlox เป็นแพลตฟอร์มที่ให้บริการ Backend-as-a-Service (BaaS) ที่ช่วยในการพัฒนาแอปพลิเคชันที่มีฟีเจอร์ต่างๆ เช่น การส่งข้อความ การโทรแบบเสียงและวิดีโอ รวมถึงการจัดการข้อมูลผู้ใช้ โดย QuickBlox มีการสนับสนุนเทคโนโลยี WebRTC อย่างเต็มที่ ซึ่งทำให้สามารถสร้างแอปพลิเคชันที่มีฟีเจอร์การสื่อสารแบบเรียลไทม์ได้อย่างรวดเร็วและง่ายดาย
WebRTC (Web Real-Time Communication) เป็นเทคโนโลยีที่ช่วยให้เบราว์เซอร์และแอปพลิเคชันมือถือสามารถทำการสื่อสารแบบเสียงและวิดีโอโดยตรง (peer-to-peer) โดยไม่ต้องผ่านเซิร์ฟเวอร์กลาง ซึ่ง QuickBlox ได้นำ WebRTC มาใช้เพื่อให้บริการ : Video Calling and Conferencing: QuickBlox สนับสนุนการโทรแบบวิดีโอระหว่างผู้ใช้หรือการประชุมแบบกลุ่ม ซึ่งเป็นไปได้ด้วยการใช้ WebRTC
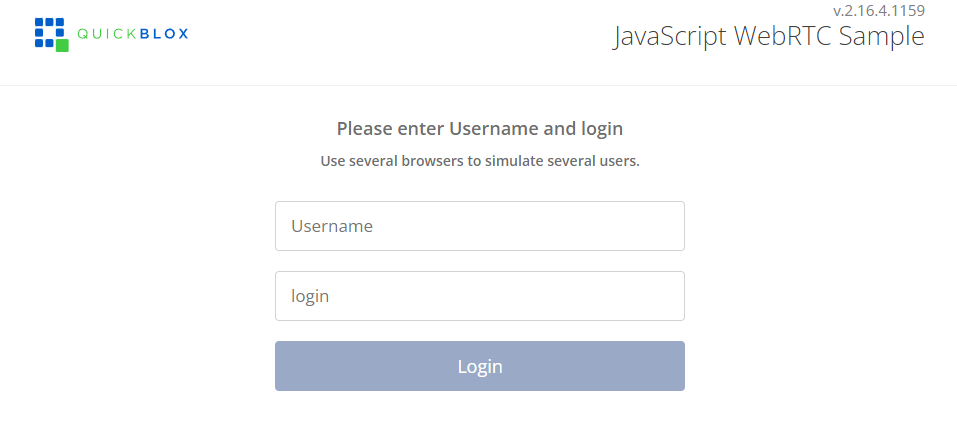
เนื่องจากเวลาจำกัดเลยใช้ WEBRTC ที่เป็น Demo ของทาง Quickblox ในการวิดีโอคอล ซึ่งให้ผลที่ดี
ทดสอบ Performance ทำให้มีดีเลย์ประมาณ < 1 วินาที ซึ่งยอมรับได้
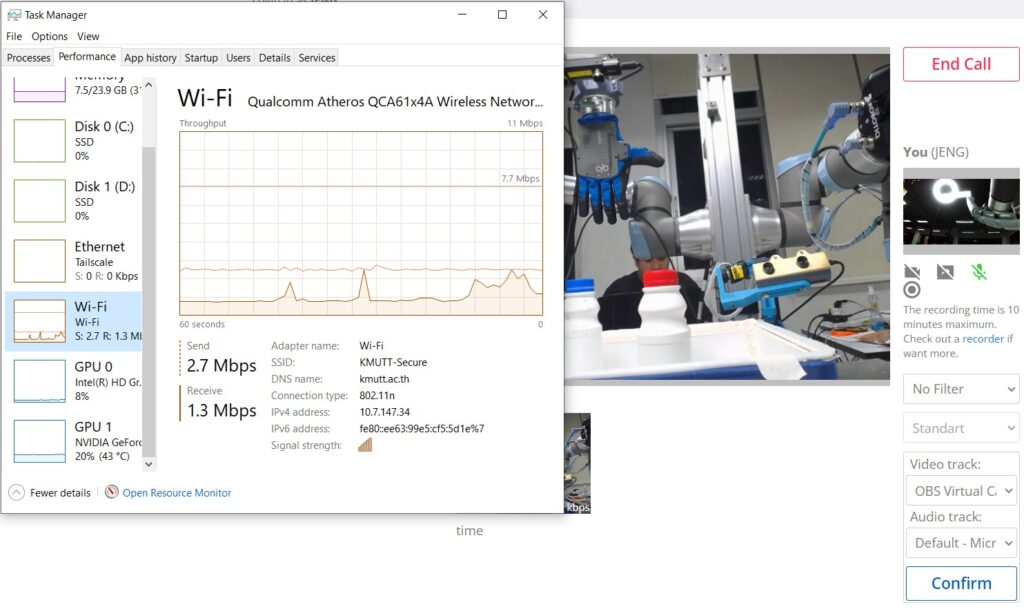
ทั้งสองเครื่องต้องทำการ Run _Code JavaScript ของ Quickblox
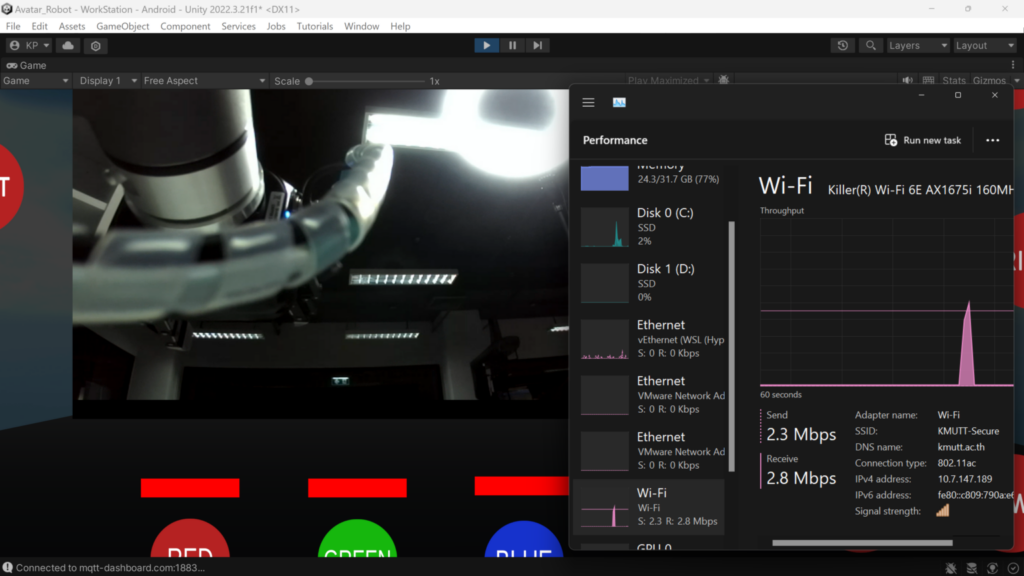